Routing in React can be a breeze with the right tools. But what if you want to add a layer of complexity, quite literally? In this post, we'll explore how to use layers in React to create dynamic, user-friendly interfaces. We'll be using react-router
and react-router-dom
for routing, and grommet
for our UI components.
Why Layers?
Imagine a scenario where you want to display additional information without navigating away from the current page. Layers, similar to modals, allow you to overlay components on top of a mounted container. This can be especially useful for showing detailed information without overwhelming the user or breaking the flow of the application.
The Challenge
Let's consider a music application. The main dashboard displays a list of artists. Clicking on an artist's name should open their profile in a layer on top of the dashboard. Now, what if you want to share a direct link to an artist's profile? The user should be able to view the artist's profile without first navigating to the dashboard. This is where our routing challenge begins.
A Dynamic Solution
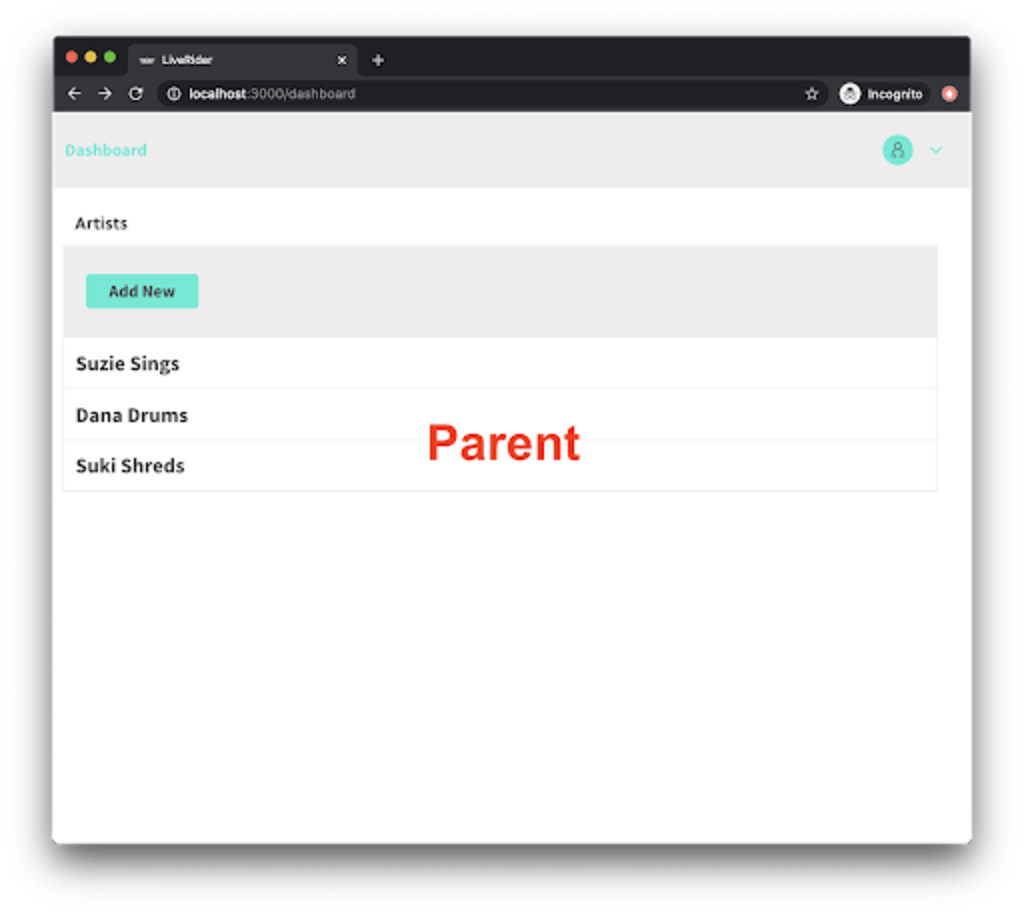
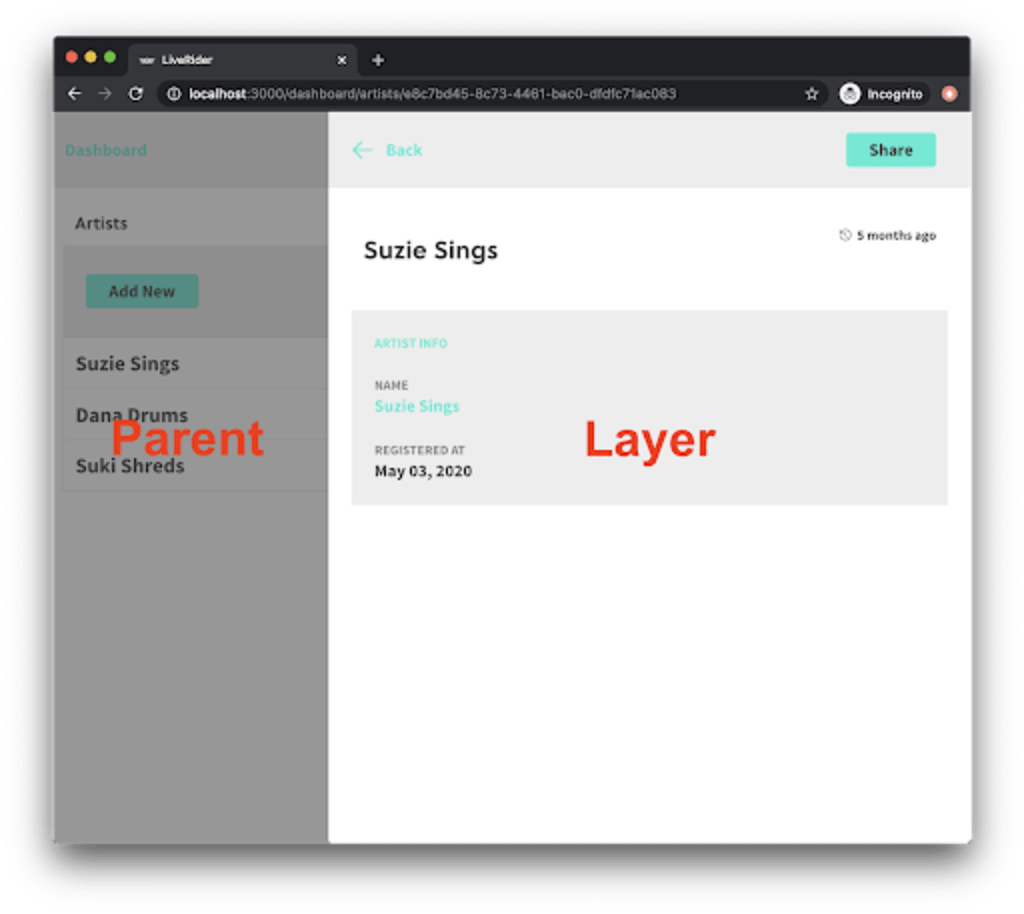
Here's how we can achieve this:
- Artist Profile Layer: The visibility of the layer is controlled by props (
showLayer
andsetShowLayer
). When the layer is visible, it displays the artist's profile.
const ArtistProfile = ({ showLayer, setShowLayer }) => (
{showLayer && (
<Layer
onClickOutside={() => setShowLayer(false)}
onEsc={() => setShowLayer(false)}
>
<ArtistProfile />
</Layer>
)}
)
- Dynamic Routing: We need to set up our routes in a way that allows the dashboard to remain visible behind the artist profile layer. This is achieved by dynamically setting the
location
in ourSwitch
based on whatbackground
is set to.
export default function Routes() {
const location = useLocation()
const background = location.state && location.state.background
return (
<>
<Container>
<Switch location={background || location}>
<PrivateRoute exact path="/dashboard" component={Dashboard} />
</Switch>
<PrivateRoute
path="/dashboard/artist/:uid"
component={ArtistProfile}
key={Date.now()}
/>
</Container>
</>
)
}
- Navigation: When navigating from the Dashboard to an artist's profile, we set the background as the current location (
/dashboard
). This ensures that the dashboard remains mounted and visible behind the profile layer.
history.push({
pathname: `/dashboard/artist/${artist.uid}`,
state: { background: location },
})
Conclusion
With this setup, we can now share direct links to artist profiles, and users will be greeted with a dynamic layer on top of the dashboard. This approach enhances user experience by providing detailed information without disrupting the application's flow. Whether you're building a music app or any other platform, layers in React can be a game-changer for your UI/UX design.